In today’s digital landscape, web accessibility has evolved from being a nice-to-have to becoming a legal and moral responsibility. It’s not just about following regulations — it’s about ensuring that everyone can navigate and use the web, regardless of their abilities.
As developers, it’s easy to get caught up in delivering features and perfecting the design. But in the world of e-commerce, especially with platforms like Shopware, ignoring accessibility can alienate potential customers and even lead to legal risks. Accessibility should be as fundamental to your development process as code quality and performance.
In this article, we’ll explore how you can test your Shopware plugins for WCAG compliance using AXE and Cypress. These tools can help you catch accessibility issues early, ensuring your plugins are not only functional but also inclusive.
Our Goal
We want to automatically test our plugins for accessibility issues by using testing frameworks such as Cypress or Playwright.
There is never a 100% guarantee when testing this way, but at least the most common issues are prevented. Just imagine running these tests in your CI pipeline next to your E2E tests. Isn't that great to have your pipeline automatically fail when you introduce an accessibility issue?
And have you ever used a Test Management Software such as TestRail before? What happens if you even add these type of tests to your test suite and strategically automate them? Spoiler - you end up adding a totally new level of quality to your plugins by creating structured and focused accessibility tests.
Read more about Automating your tests with Cypress and TestRail.
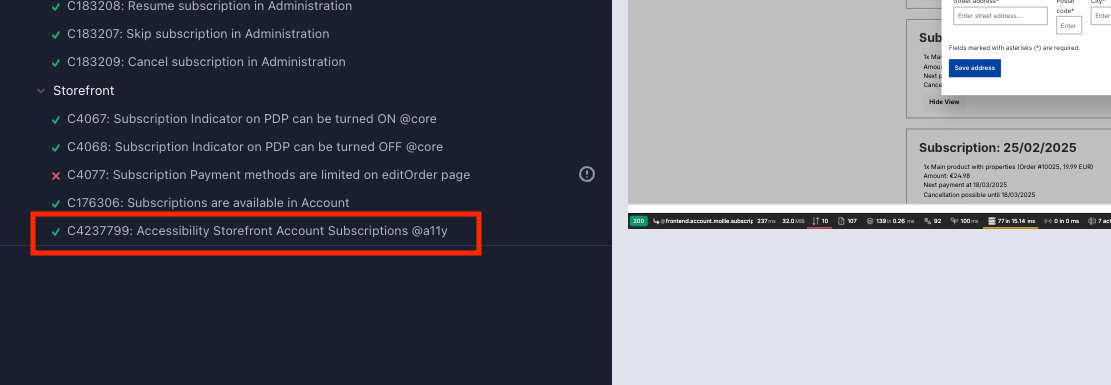
Let's first talk about some basics of accessibility.
What is WCAG?
The Web Content Accessibility Guidelines (WCAG) are a set of internationally recognized standards developed by the W3C (World Wide Web Consortium) to ensure web content is accessible to all users, including those with disabilities.
WCAG is built around 4 key principles, ensuring that content is:
- Perceivable: Users must be able to see, hear, or otherwise sense the content.
- Operable: Users must be able to use buttons, links, and other controls.
- Understandable: Content and how to use the website must be easy to understand.
- Robust: The website must work well with different devices and assistive tools.
WCAG provides different conformance levels: A (lowest), AA (mid-range), and AAA (highest). Most organizations aim for WCAG 2.1 AA compliance as it covers essential accessibility needs without being overly restrictive.
What is A11y?
When you read about accessibility, you might see terms like A11y. This is nothing to be afraid of, it just means accessibility.
The term is derived from the word “accessibility” by taking the first and last letters and the number of characters in between (11).
For me, it's also a great shortcut to e.g. enhance my Cypress tests with a tag "a11y" to quickly identify and run my accessibility tests in my test suite.
What is ARIA?
ARIA is another term you will come across when talking about accessibility. ARIA stands for Accessible Rich Internet Applications. It is a set of attributes added to HTML to make web content and applications more accessible to people with disabilities, especially those using assistive technologies like screen readers.
Here is a sample of an ARIA tag for the label of a button:
<button aria-label="Close">X</button>
What ARIA does:
- Improves Accessibility: Helps assistive technologies understand dynamic content and complex UI elements.
- Enhances Interaction: Provides extra information about elements like sliders, menus, and tabs that standard HTML doesn’t cover.
- Describes Roles and States: ARIA attributes define roles (e.g., role="button"), states (e.g., aria-disabled="true"), and properties (e.g., aria-label="Close").
What is Axe?
Axe is an open-source accessibility testing engine developed by Deque Systems. It helps developers, testers, and designers automatically detect accessibility issues in web applications. Axe integrates seamlessly into various development tools and environments, making accessibility testing part of everyday workflows.
Key Features of Axe:
- Automated Testing: Quickly identifies common accessibility violations.
- Integration Friendly: Works with tools like Chrome DevTools, Cypress, Selenium, and various CI/CD pipelines.
- Customizable: Allows fine-tuning of rules to fit project-specific needs.
- Detailed Reports: Provides clear descriptions of issues with actionable solutions.
Now that we have learned the terms and tools, let's see how we can use them to test our Shopware plugins.
Testing Shopware Plugins
Let's imagine you already have a Shopware plugin.
The first thing we want to add, is an automated testing framework such as Cypress. Therefore, we want to install Cypress along with the cypress-axe plugin.
There are also plugins for Playwright or Selenium, so you have the choice to use the one you are most comfortable with. In this tutorial, we will focus on Cypress.
1. Install Cypress and Axe
First, install the necessary dependencies:
npm install --save-dev cypress cypress-axe
If you don't know how to set up Cypress, you can follow the official documentation on their website.
To enable the Axe plugin, we just need to add the following code snippet to our cypress/support/e2e.js file.
import 'cypress-axe';
2. Write your first accessibility test
Writing an accessibility test is straightforward with Cypress and Axe.
We first need to plan what we try to achieve with our test.
Testing accessibility means we test something the user can see or interact with. Not every plugin modifies the storefront of Shopware.
If you have such a plugin, think about the parts that your plugin changes. Maybe some blocks of existing pages are modified, or maybe even a whole new page is added to the Shopware Storefront.
When we write a test in Cypress, we first need to visit the page (as always). Once we are on our page, we need to run the command cy.injectAxe(). This will inject the accessibility engine into our application and needs to be done on every page we want to test. After this is done, we can already run our tests with cy.checkA11y().
it('Test Home Page Accessibility', () => {
cy.visit('/');
// have axe inject itself using javascript
cy.injectAxe();
// start the tests
cy.checkA11y();
});
Wow, that was easy, right? We have successfully tested the full home page of Shopware for accessibility issues.
Unfortunately, this will also test the header, footer, and all other parts of the Shopware Storefront. And we all know, that your plugin might not be responsible for all of these parts, right?
We can easily limit the scope of our tests by specifying a selector. Let’s say we have a custom page where we want to test only the main content, excluding the header, footer, and sidebar of Shopware.
it('Tests accessibility of the plugin account page', () => {
cy.visit('/account/my-page');
cy.injectAxe();
// start tests only for the main content
cy.checkA11y('.account-content-main');
});
Now start your Cypress tests, and you should already see the first results for your plugin. In our case, we have an error, because we have specified a h3 title, but h2 is missing on our page. So we have a valid h1 and a h3, but no h2.
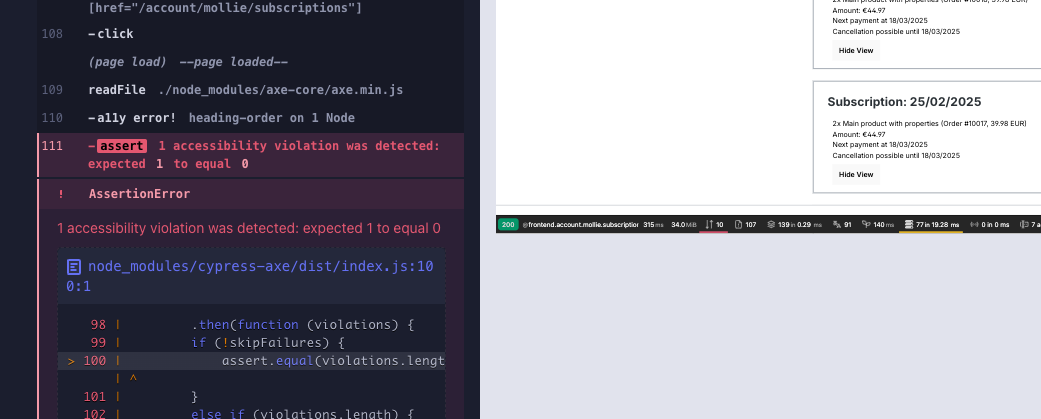
We can fix our issue by just using h2 instead of h3 in our case. After we have cleaned up our code, we can run our tests again and Cypress will show us a success message.
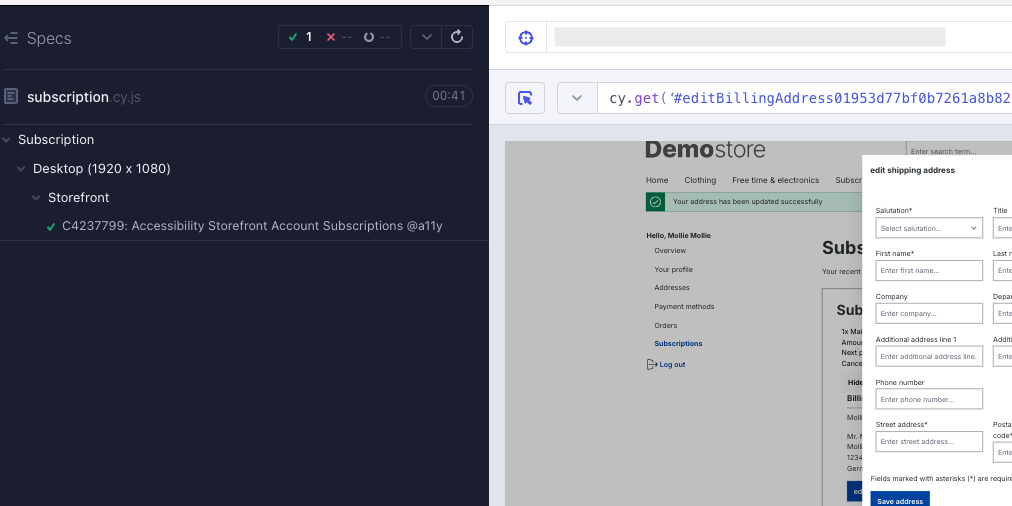
That's it! You have successfully written your first accessibility test for your Shopware plugin.
Common issues you might encounter
When you encounter an issue, and you don't immediately know how to fix it, you can always refer to the Axe documentation or just google the error message.
Here are a few common issues you might encounter:
- aria-valid-attr-value: Invalid ARIA attribute values. Make sure ARIA attributes like
aria-expanded
oraria-hidden
have valid boolean strings ("true"
or"false"
). - button-name: Buttons without accessible names. Use visible text or
aria-label
to resolve this. - color-contrast: Insufficient contrast between text and background colors.
Conclusion
With the latest laws and regulations, accessibility is no longer optional. While not every shop is required to comply with WCAG, it’s essential to make at least your Shopware plugins accessible to everyone.
Axe is a great tool, and there are lots of different configuration options, like rules, checks, reports and more, that can help you to get even more out of it.
Cypress and Axe might not be a 100% guarantee and can never fully test everything, but they are a great start to catch common issues early in your development process.
Adding these kinds of tests to your existing CI pipeline can help you ensure that your plugins are not only functional but also inclusive.
Links: